How To Improve Your App’S Performance And Reduce Load Time
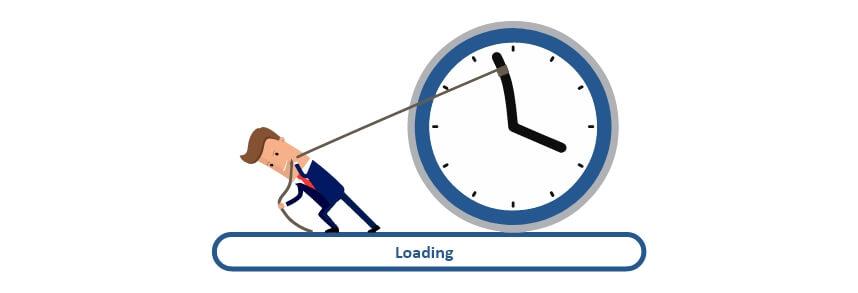
In today’s fast-paced digital environment, users expect mobile apps to be fast, reliable, and responsive. A slow app not only frustrates users but also increases the risk of churn and negative reviews. Improving your app’s performance and reducing its load time are crucial for maintaining user satisfaction and achieving business success. This blog explores practical strategies and best practices to enhance your app’s performance and ensure it runs smoothly on any device.
1. Optimize Code for Efficiency
a) Minimize Code Complexity
Write clean and efficient code by following best practices such as modular programming, avoiding unnecessary loops, and removing redundant code. Refactor your code regularly to eliminate inefficiencies.
b) Lazy Loading
Load only the necessary components initially, deferring the loading of non-critical resources until they are needed. This reduces the initial load time and improves perceived performance.
Example:
const loadHeavyComponent = () => import('./HeavyComponent');
c) Use Efficient Algorithms
Choose algorithms that are optimized for your app’s specific requirements. Opt for data structures and algorithms with lower time and space complexity to improve performance.
2. Compress Assets
a) Image Optimization
Compress images using tools like TinyPNG or ImageOptim. Use modern image formats like WebP, which provide smaller file sizes without compromising quality.
Example:
<img src="image.webp" alt="Optimized Image">
b) Minify Resources
Reduce the size of CSS, JavaScript, and HTML files by minifying them. Tools like UglifyJS, CSSNano, and HTMLMinifier can automate this process.
c) Optimize Fonts
Use modern, web-optimized font formats like WOFF2. Include only the font weights and styles required for your app.
3. Implement Caching
a) Use HTTP Caching
Leverage HTTP caching headers to store frequently accessed resources locally. This reduces server requests and speeds up load times for returning users.
Example (Apache):
<IfModule mod_expires.c>
ExpiresActive On
ExpiresByType image/jpeg "access plus 1 year"
</IfModule>
b) Implement In-App Caching
Store API responses, images, and other data locally on the device using caching libraries. This minimizes network requests and improves performance.
Example (React Native):
import AsyncStorage from '@react-native-async-storage/async-storage';
const saveData = async (key, value) => {
await AsyncStorage.setItem(key, JSON.stringify(value));
};
4. Reduce Network Latency
a) Optimize API Calls
Minimize the number of API requests by combining multiple requests into a single batch. Use pagination to load large datasets incrementally.
Example:
GET /api/items?page=1&pageSize=20
b) Use a Content Delivery Network (CDN)
CDNs cache content on servers worldwide, ensuring faster delivery of resources to users based on their geographical location.
c) Enable HTTP/2
Switch to HTTP/2 to take advantage of multiplexing, which allows multiple requests to be sent over a single connection, reducing latency.
5. Leverage Multithreading and Asynchronous Operations
a) Background Tasks
Offload heavy computations to background threads to prevent blocking the main UI thread. Use multithreading libraries or frameworks to achieve this.
Example (Android):
Thread {
// Background task
}.start()
b) Asynchronous Data Loading
Fetch data asynchronously to keep the app’s UI responsive.
Example:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data));
6. Monitor and Optimize Database Performance
a) Index Your Database
Add indexes to frequently queried fields to speed up database operations. However, avoid over-indexing, as it can slow down write operations.
b) Use a Local Database
For offline capabilities, use lightweight local databases like SQLite or Room. Synchronize data with the server periodically.
c) Optimize Queries
Write efficient queries and avoid fetching unnecessary data. Use query optimization tools to analyze and improve performance.
Example:
SELECT name, age FROM users WHERE active = 1;
7. Enhance Rendering Performance
a) Reduce Repaints and Reflows
Minimize changes to the DOM to avoid costly repaints and reflows. Use techniques like virtual scrolling for lists and lazy rendering for components.
b) Use Hardware Acceleration
Leverage the GPU for animations and transitions to ensure smoother performance.
Example (CSS):
.element {
transform: translateZ(0);
}
c) Implement Virtual DOM
For web apps, use frameworks like React or Vue.js that implement a virtual DOM to optimize UI rendering.
8. Optimize Startup Time
a) Reduce App Bundle Size
Use code-splitting to load only the necessary modules initially. Tools like Webpack and Rollup can help with this.
b) Preload Critical Resources
Preload assets and scripts essential for the initial screen to speed up rendering.
Example:
<link rel="preload" href="styles.css" as="style">
c) Show a Splash Screen
Display a splash screen during startup to improve perceived performance while the app loads in the background.
9. Conduct Performance Testing and Monitoring
a) Use Profiling Tools
Leverage tools like Android Studio Profiler, Xcode Instruments, or Lighthouse to analyze app performance and identify bottlenecks.
b) Monitor Real-Time Performance
Integrate performance monitoring solutions like Firebase Performance Monitoring or New Relic to track metrics like load time, crash rates, and network latency.
c) User Feedback
Collect user feedback to identify performance issues that may not be evident during testing.
10. Ensure Regular Updates and Maintenance
a) Address Bugs and Issues
Fix reported issues promptly to maintain performance and user satisfaction.
b) Stay Updated with Technology
Keep libraries, frameworks, and dependencies updated to leverage the latest performance improvements.
c) Test on Multiple Devices
Ensure your app performs well across a range of devices and operating systems by testing it on emulators and real devices.
Conclusion
Improving your app’s performance and reducing load time is a continuous process that requires attention to detail and a proactive approach. By implementing the strategies outlined above, you can ensure that your app delivers a fast, smooth, and engaging experience for users. Prioritize optimization at every stage of development to stay ahead in the competitive mobile app landscape.